In the Bluesky (social media platform) web interface, post dates are shown relative to the current date/time. To see the actual post time, you have to hover over the date.
I’ve written a Tampermonkey script that automatically expands the displayed time to be the actual date/time instead of time since post.
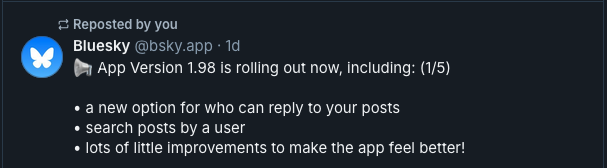
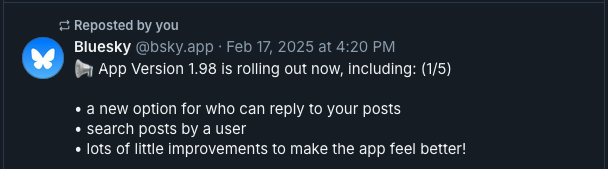
Here’s the script:
// ==UserScript== // @name Bluesky Post Time Expander // @namespace http://tampermonkey.net/ // @version 1.0 // @description Show full date instead of time since post // @author Shawn Hooper // @match *://bsky.app/* // @grant none // ==/UserScript== (function() { 'use strict'; // Function to replace div content with the value from aria-label function replaceContentWithAriaLabel() { // Select all divs with an aria-label attribute const elements = document.querySelectorAll('a[data-tooltip][role="link"]:not([data-tampermonkey])'); console.log(elements); elements.forEach(element => { const ariaLabel = element.getAttribute('data-tooltip'); element.textContent = abbreviateMonth(ariaLabel); // Replace content with aria-label value element.setAttribute('data-tampermonkey', 1); }); } function abbreviateMonth(inputString) { const monthMap = { January: 'Jan', February: 'Feb', March: 'Mar', April: 'Apr', May: 'May', June: 'Jun', July: 'Jul', August: 'Aug', September: 'Sep', October: 'Oct', November: 'Nov', December: 'Dec' }; // Create a regular expression to match full month names const monthRegex = new RegExp(`\\b(${Object.keys(monthMap).join('|')})\\b`, 'gi'); // Replace full month names with their abbreviations return inputString.replace(monthRegex, (match) => monthMap[match]); } // Set up MutationObserver to watch for changes in the document function observeMutations() { const targetNode = document.body; const config = { childList: true, subtree: true }; const callback = function(mutationsList) { for (const mutation of mutationsList) { if (mutation.type === 'childList') { // Call the function to replace content console.error('Content Changed!'); replaceContentWithAriaLabel(); } } }; const observer = new MutationObserver(callback); observer.observe(targetNode, config); } // Run the function initially to replace existing content replaceContentWithAriaLabel(); // Start observing for dynamic content changes observeMutations(); })();